Popup Form is a common web element. If you want, you can create a Popup Form with HTML and CSS only. In many places on the website, we see Popup Login Form, Popup Registration Form, and Popup Email Subscribe Form.
If you want to add this type of popup window to your project then this tutorial is for you. Here we will create a Popup Login Form using HTML, CSS, and javascript.
There are different types of Popup Form CSS. In some cases, the form is opened automatically and in some cases, the box has to be opened manually with a button.
Popup Form in HTML CSS
In this tutorial, I will discuss how to create a Popup Form using HTML CSS JavaScript. This design was originally created in the form of a login form. Simply put, this is a pop-up login form.
You will find many such tutorials on the Internet. But here I am giving you everything a Beginners need. Here we have given a step-by-step tutorial, live preview, and source code.
See the Pen Untitled by Shantanu Jana (@shantanu-jana) on CodePen.
We all know that in the case of a popup window, only one button or link is found on the web page. A button can be found in the case of this project.
After clicking on that button, you will see this pop-up login form. The popup box contains a cancel button that will hide.
Since this is a login form, there is room to input your email ID and password. It comes with a submit button and some basic text.
How to Create a Popup Form in HTML
You must have some idea about HTML and CSS to create a popup form on a button click. In this tutorial, we will cover .....
Here HTML is used to add all the basic information. It is designed by CSS and activates the JavaScript popup button.
Step 1:
Create a button to open the Popup form
A button was first created using the following HTML and CSS codes. Clicking on this button can open a popup from HTML.
The size of the button depends on the padding: 15px 50px and the background color is white. transform: using translate (-50%, -50%) This button is placed in the middle of the webpage.
Step 2:
The basic structure of HTML Popup Form
Now we need to create a pop-up form. Basically, first, we will create a popup box. Then I will add all the information in that box.
I have used the width of this box: 350px, height: 430px. However, you can adjust it according to your needs.
Here 'transform:' has been removed -150% along the y-axis using translate (-50%, -150%). As a result, we will not be able to see this Simple Popup Form under normal circumstances.
Now, this Popup form HTML has been implemented using the 'active' tag. The instructions here are transform: translate (-50%, -50%) when you click on the pop-up button. This HTML popup login form can be seen in the middle of the webpage.
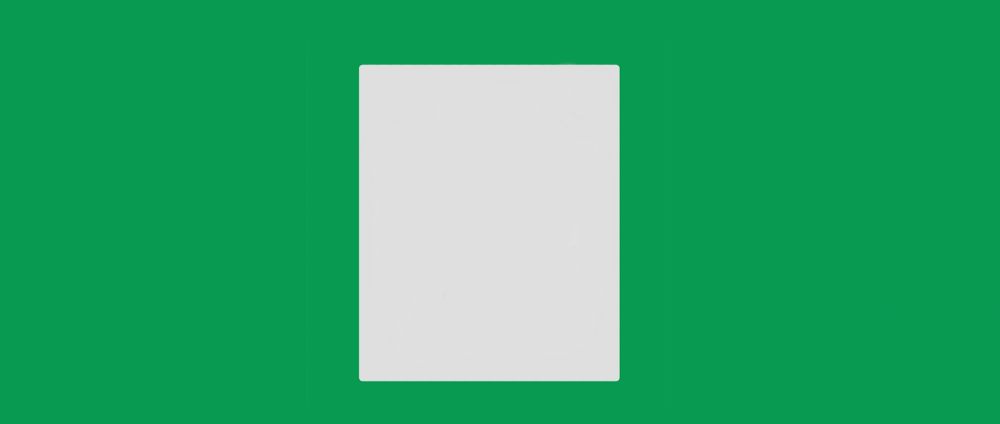
Step 3:
Cancel button of the modal popup form
Now we need to create the cancel button. This cancel button will help to hide the Popup form HTML CSS.
Step 4:
Information of popup login form
Now all the information of the login form has been added in this popup form on button click. A heading, two input boxes, a submit button, and some basic text are added here.
The following CSS has been used to design the login button in the popup login form. Button size depending on padding: 15px 40px. I have used blue as the background color and the text color is white.
Step 5:
Activate the popup window using JavaScript
The Popup Login Form is fully designed. Form popup must now be executed using just one line of JavaScript.
You must comment on how you like this popup window. So far I have shared tutorials on creating different types of forms using popup boxes. All the code of this Simple Popup Form HTML is in the download button below.